Learn to code with confidence
Free, interactive coding tutorials to help you master programming. From beginner to advanced, learn at your own pace with hands-on examples.
Featured Courses
Discover our most popular programming courses and start learning today.
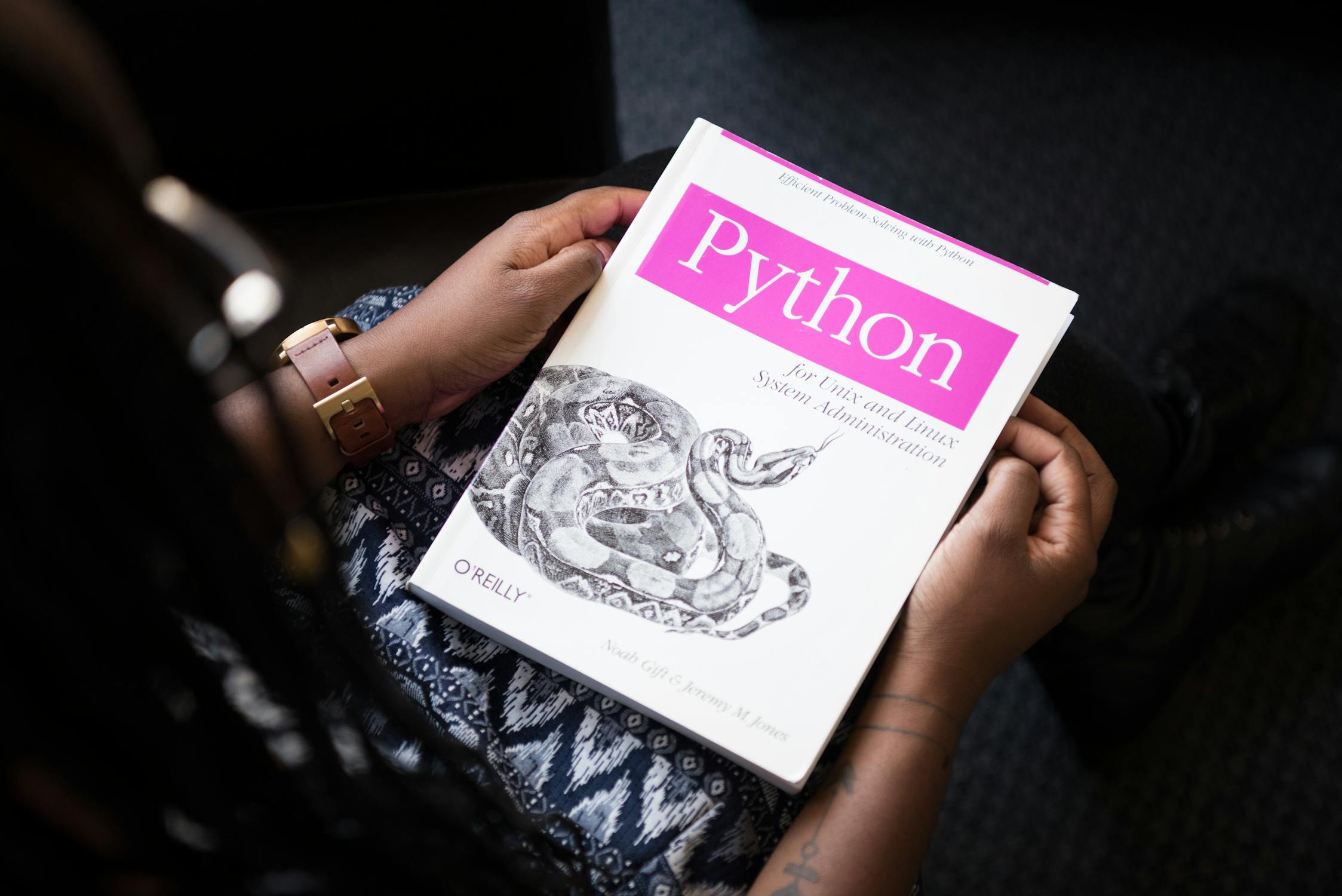
Python for Beginners
Start your programming journey with Python, the most beginner-friendly language.

JavaScript Essentials
Learn the language of the web and build interactive websites.
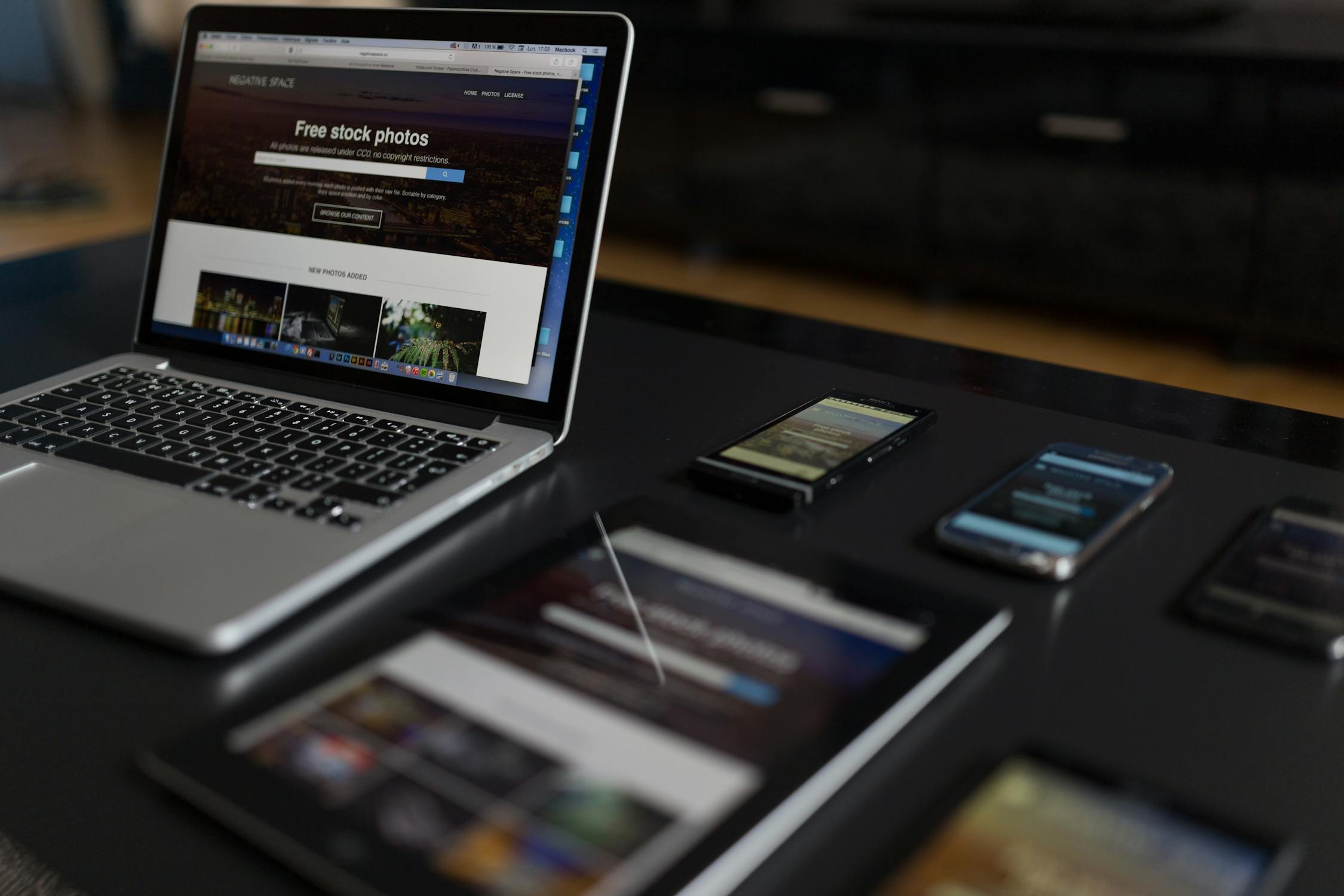
Web Development Bootcamp
Comprehensive course covering HTML, CSS, JavaScript, and modern frameworks.
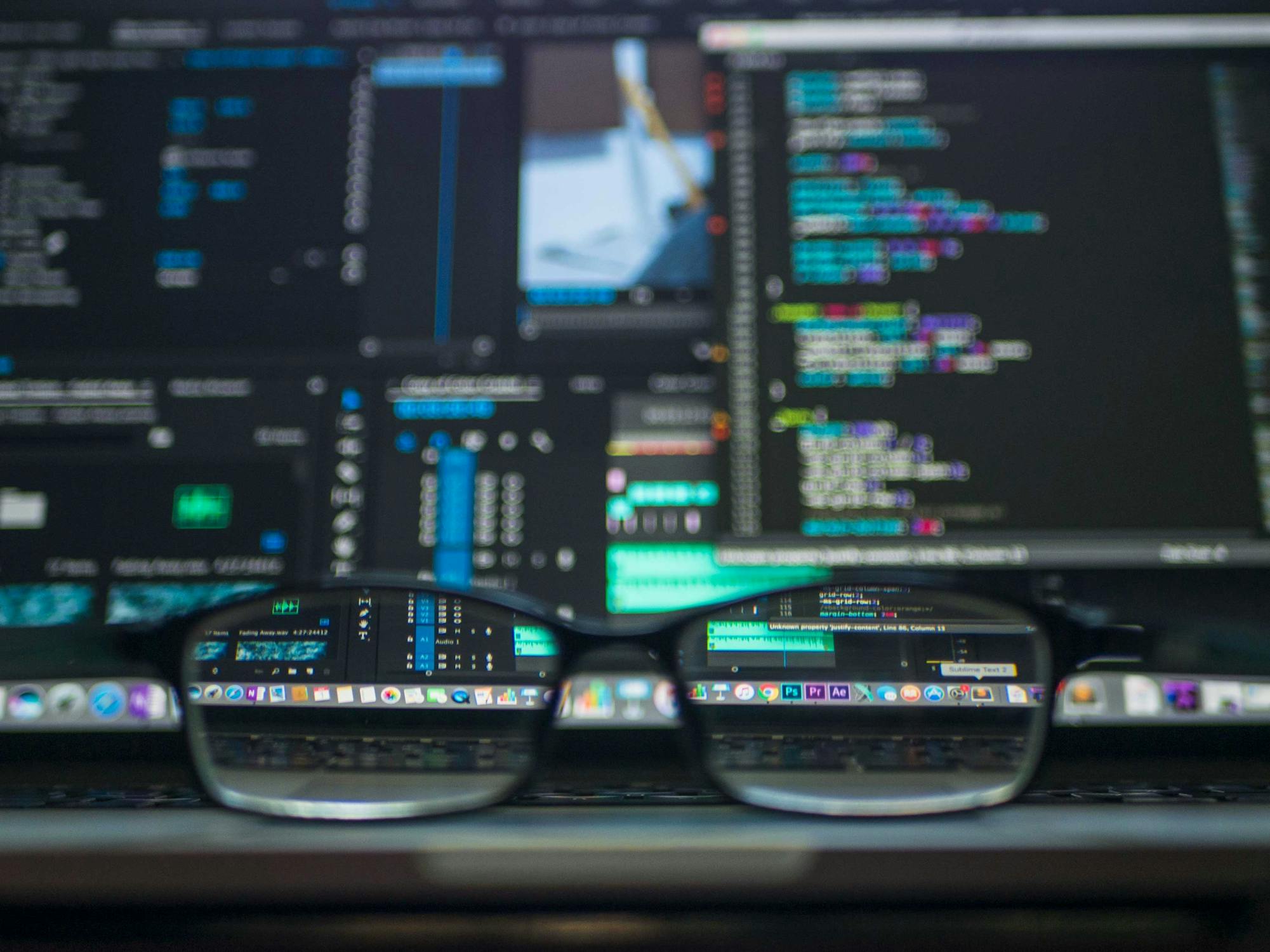
Data Science with Python
Learn how to analyze data, create visualizations, and build predictive models.
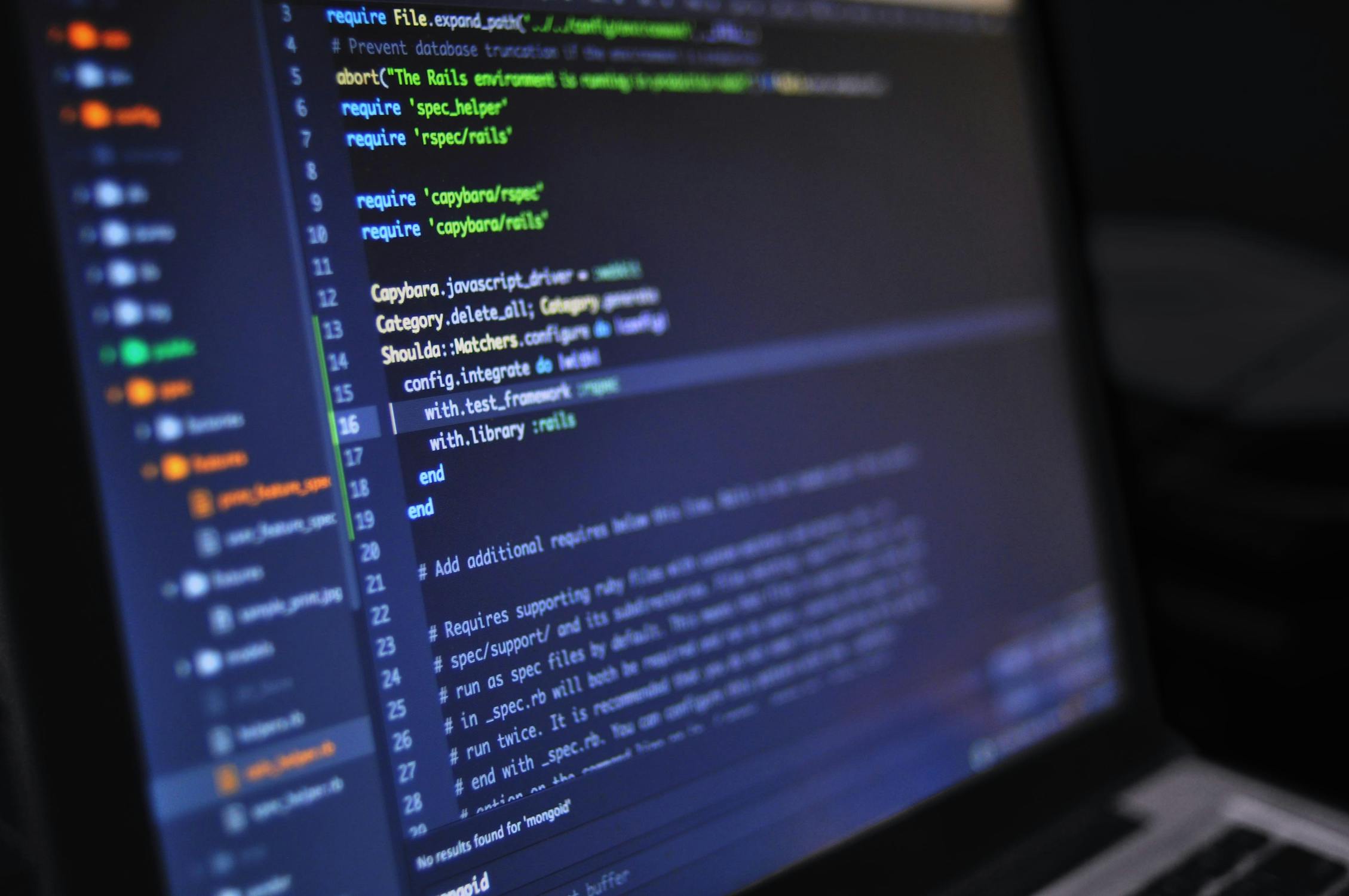
Java Programming Masterclass
Master Java programming from the basics to advanced concepts.
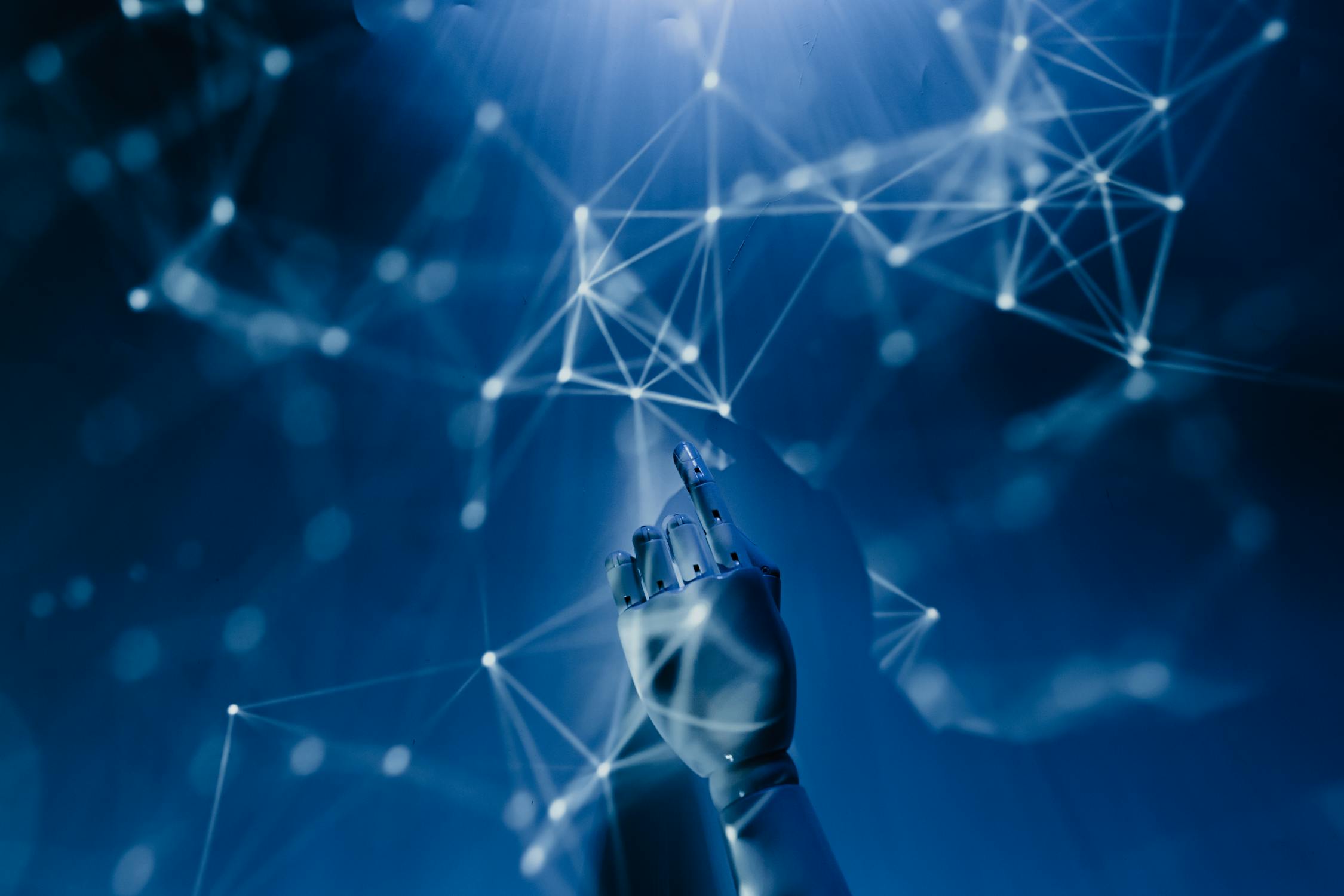
Data Structures & Algorithms
Introduction to fundamental data structures and algorithms for efficient programming.
Why Choose PrologiCode
Our platform is designed to provide the best learning experience for programmers at all levels.
Comprehensive Tutorials
Access detailed tutorials for multiple programming languages, frameworks, and concepts, from beginner to advanced.
Interactive Code Playground
Practice coding directly in your browser with our interactive code editor and real-time compilation.
Hands-on Exercises
Reinforce your learning with practical exercises and projects that build real-world skills.
AI-Powered Assistance
Get smart suggestions, error explanations, and coding help through our AI coding assistant.
Progress Tracking
Monitor your learning journey with detailed progress tracking and achievement badges.
Community Support
Connect with fellow learners, ask questions, and collaborate on coding challenges.
Choose Your Learning Path
Follow structured learning paths designed to take you from beginner to professional in your chosen field.
Web Development
Build modern, responsive websites and web applications
Java Programming
Master Java programming from fundamentals to enterprise applications
Data Science
Master data analysis, visualization, and machine learning
Mobile Development
Create apps for iOS and Android platforms
Game Development
Design and develop engaging games for various platforms
What Our Learners Say
Thousands of students have accelerated their coding journey with PrologiCode. Here's what some of them have to say.
"PrologiCode helped me transition from a non-technical role to a full-stack developer in just 6 months. The structured learning paths and practical exercises made a huge difference."
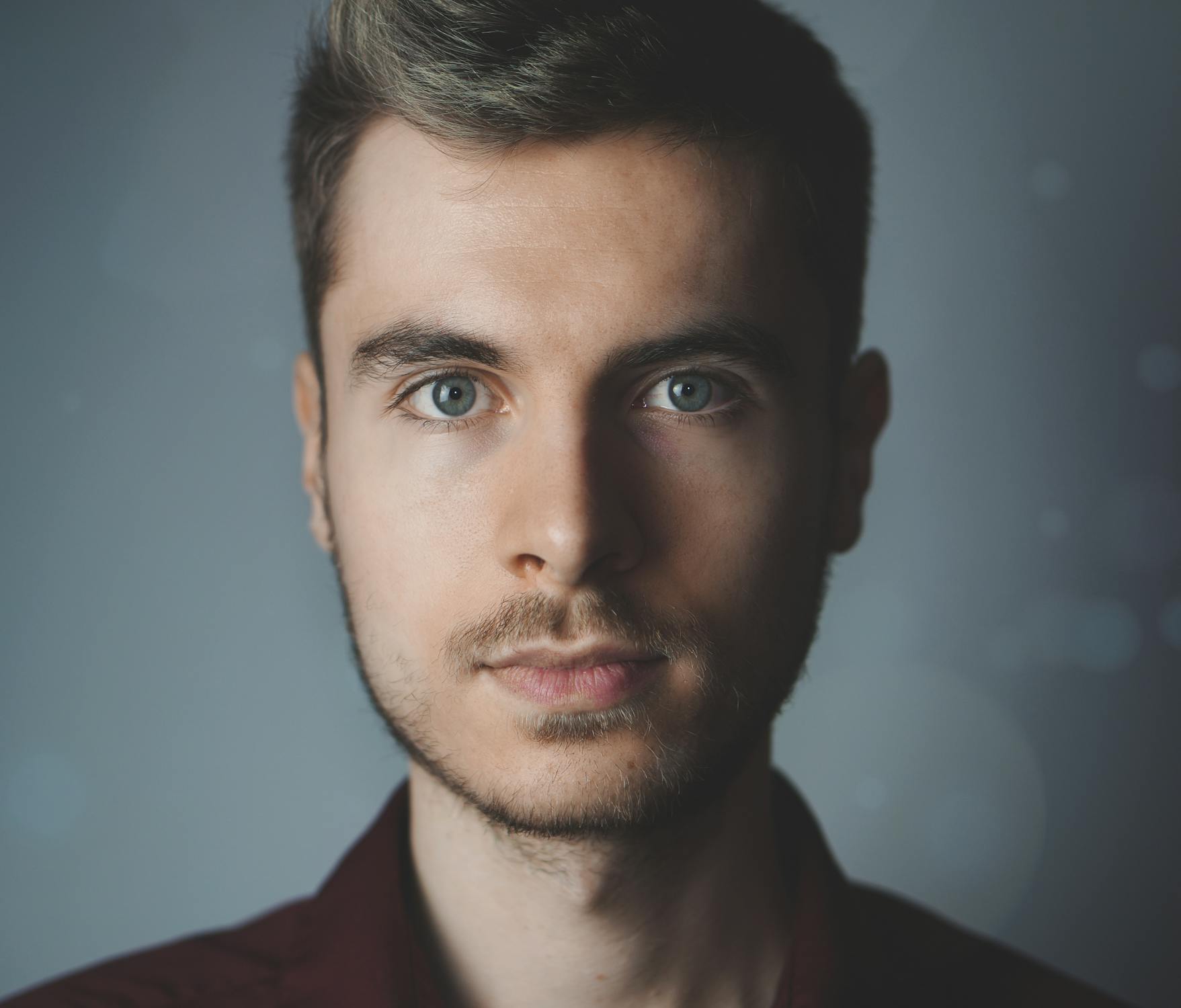
Alex Thompson
Full-Stack Developer
"The interactive code playground is fantastic! Being able to practice coding directly in the browser while learning made concepts stick much better than just reading tutorials."
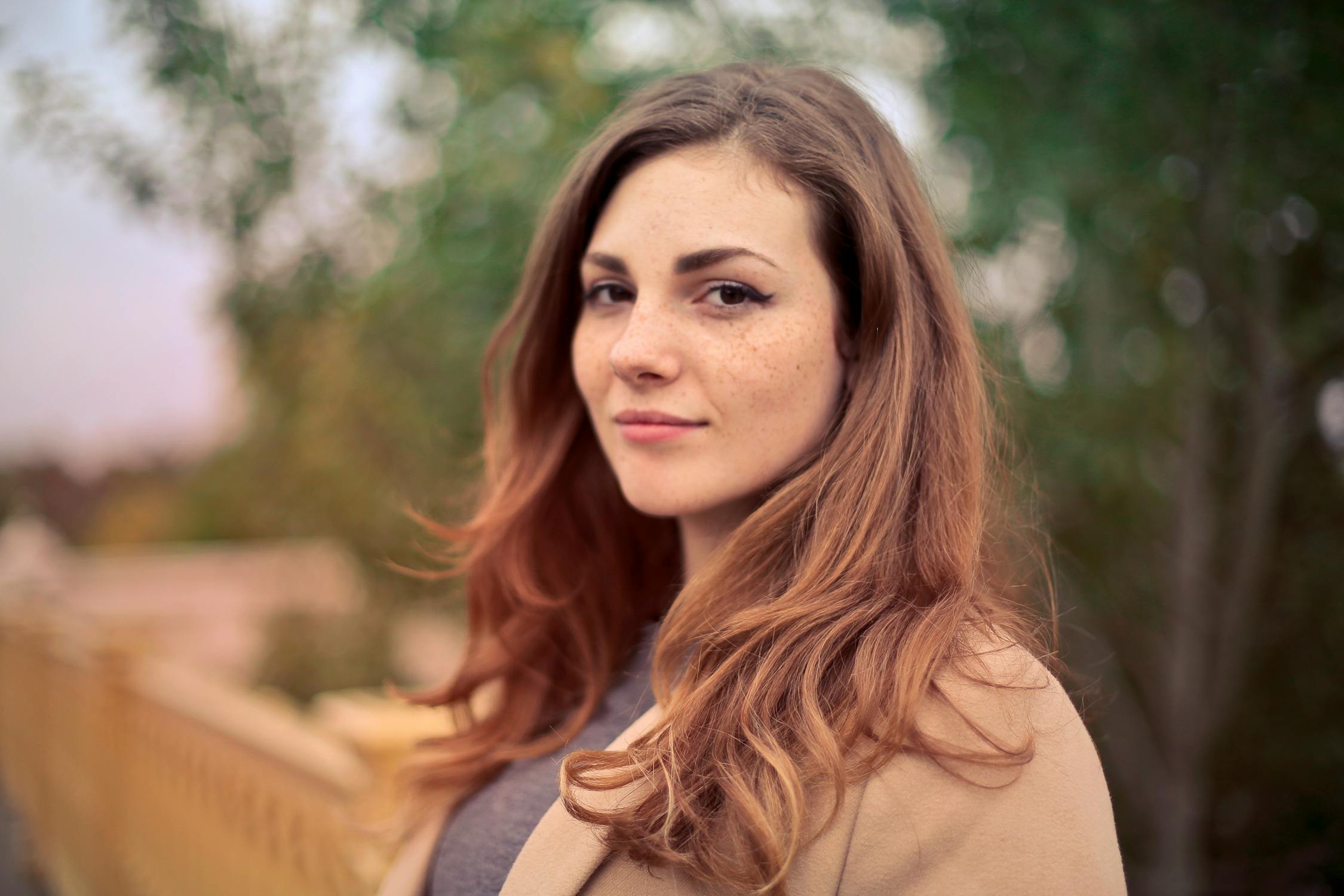
Sarah Johnson
Computer Science Student
"I've tried many coding platforms, but PrologiCode stands out for its clear explanations and well-structured content. The progression from basic to advanced topics is seamless."

Michael Chen
Software Engineer
Start Your Coding Journey Today
Join thousands of learners who have transformed their careers through coding. All tutorials are free and accessible to everyone.